YouTube Comment Picker Using Video URL Step by Step in PHP
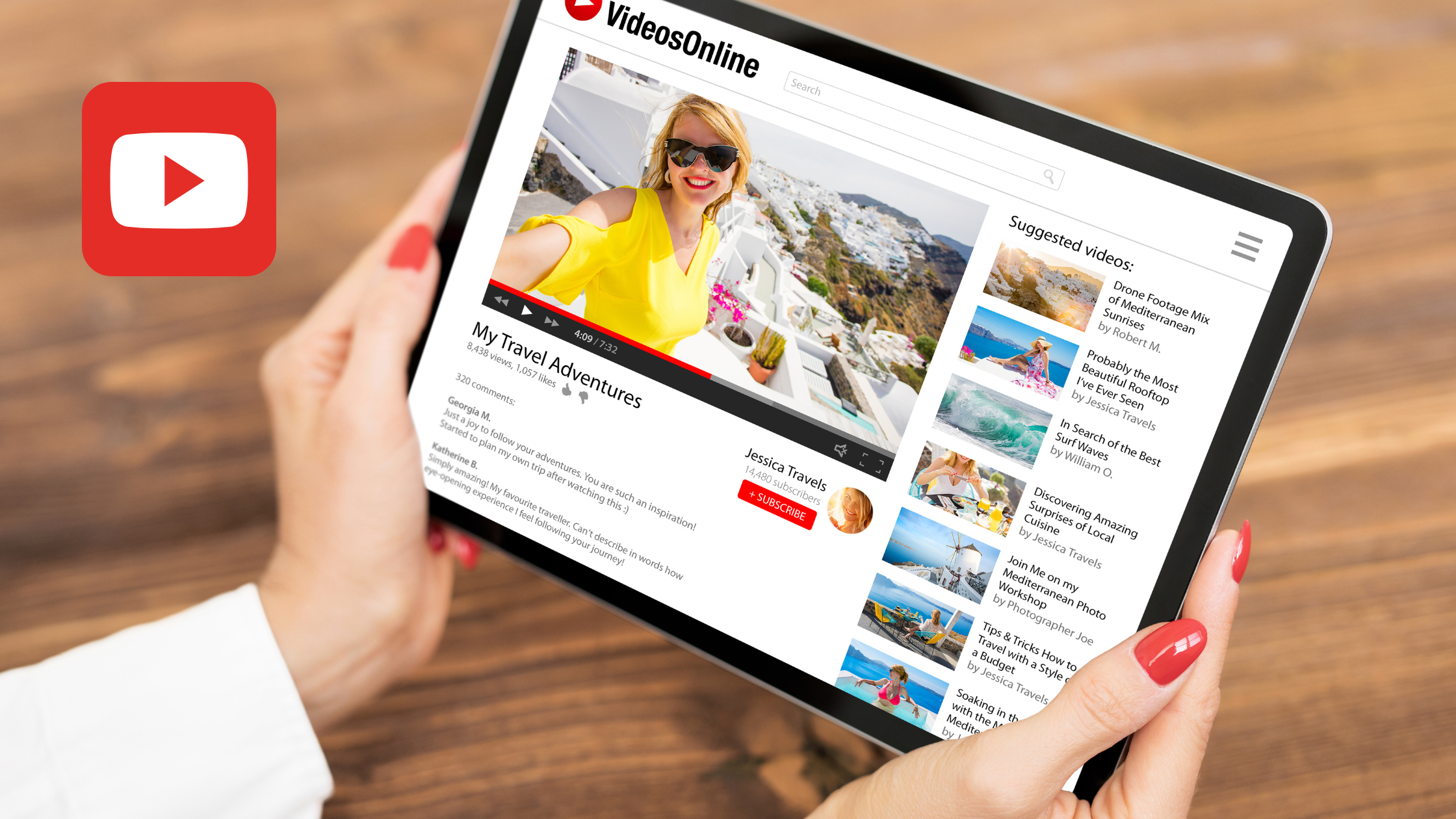
In this process will guide you how you can obtaining the YouTube API key and use for YouTube video comment picker step by step:
1. Create a Google Account: If you don’t have one already, create a Google account. You’ll need this to access the Google Developers Console.
2. Access Google Developers Console: Go to the [Google Developers Console](https://console.developers.google.com/) and sign in with your Google account.
Create a New Project: Once logged in, create a new project by clicking on the “Select a project” dropdown menu at the top and then selecting “New Project”. Give your project a name and click on “Create”.
![]() | ![]() |
![]() | ![]() |
![]() | ![]() |
![]() | ![]() |
![]() | ![]() |
![]() | ![]() |
Get your API Key: After creating the API key, it will be displayed on the screen. Make sure to copy it and keep it secure. This key will be used to authenticate your requests to the YouTube API.
Let’s Get Start using the API for YouTube Video Comment Picker
Now you have your API key ready to use. You can start making requests to the YouTube API using this key in your applications or scripts. Make sure to follow the API documentation for the endpoints you want to access. Remember to keep your API key confidential and never share it publicly, as it could be misused. Also, monitor your usage to ensure you don’t exceed any quotas or limits imposed by YouTube.
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>YouTube Video Details</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f4f4f4;
}
.container {
max-width: 600px;
margin: 50px auto;
background-color: #fff;
padding: 20px;
border-radius: 8px;
box-shadow: 0px 0px 10px 0px rgba(0, 0, 0, 0.1);
}
h1 {
text-align: center;
color: #333;
}
label {
font-weight: bold;
color: #333;
}
input[type=”text”] {
width: 97%;
padding: 10px;
margin-top: 5px;
margin-bottom: 15px;
border: 1px solid #ccc;
border-radius: 4px;
}
input[type=”submit”] {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
border-radius: 4px;
cursor: pointer;
font-size: 16px;
}
input[type=”submit”]:hover {
background-color: #45a049;
}
.video-details {
margin-top: 20px;
}
.comment-section {
margin-top: 20px;
}
.comment {
margin-bottom: 20px;
}
.author {
font-weight: bold;
color: #333;
}
.comment-text {
margin-top: 5px;
color: #555;
}
.reply {
margin-left: 20px;
}
</style>
</head>
<body>
<div class=”container”>
<h1>YouTube Video Details</h1>
<form method=”post” action=””>
<label for=”video_url”>Enter YouTube Video URL:</label><br>
<input type=”text” id=”video_url” name=”video_url” required><br>
<input type=”submit” name=”submit” value=”Submit”>
</form>
</div>
<?php
function getYouTubeVideoID($url) {
$queryString = parse_url($url, PHP_URL_QUERY);
parse_str($queryString, $params);
if (isset($params[‘v’]) && strlen($params[‘v’]) > 0) {
return $params[‘v’];
} else {
return “”;
}
}
if(isset($_POST[‘submit’])) {
$api_key = ‘Use_Your_APi_Key’;
$video_url = $_POST[‘video_url’];
$video_id = getYouTubeVideoID($video_url);
$video_api_url = ‘https://www.googleapis.com/youtube/v3/videos?part=snippet%2CcontentDetails%2Cstatistics&id=’ . $video_id . ‘&key=’ . $api_key;
$comments_api_url = ‘https://www.googleapis.com/youtube/v3/commentThreads?part=snippet&videoId=’ . $video_id . ‘&key=’ . $api_key;
$video_data = json_decode(file_get_contents($video_api_url));
$comments_data = json_decode(file_get_contents($comments_api_url));
echo ‘<br><strong>Title: </strong>’ . $video_data->items[0]->snippet->title . ‘<br>’;
echo ‘<strong>publishedAt: </strong>’ . $video_data->items[0]->snippet->publishedAt . ‘<br>’;
echo ‘<strong>Duration: </strong>’ . $video_data->items[0]->contentDetails->duration . ‘<br>’;
echo ‘<strong>View Count: </strong>’ . $video_data->items[0]->statistics->viewCount . ‘<br>’;
echo ‘<br><strong>Comments:</strong><br>’;
foreach ($comments_data->items as $comment) {
echo ‘<strong>Author:</strong> ‘ . $comment->snippet->topLevelComment->snippet->authorDisplayName . ‘<br>’;
echo ‘<strong>Comment:</strong> ‘ . $comment->snippet->topLevelComment->snippet->textDisplay . ‘<br>’;
// Display replies
$replies_api_url = ‘https://www.googleapis.com/youtube/v3/comments?part=snippet&parentId=’ . $comment->id . ‘&key=’ . $api_key;
$replies_data = json_decode(file_get_contents($replies_api_url));
foreach ($replies_data->items as $reply) {
echo ‘ <strong>Reply Author:</strong> ‘ . $reply->snippet->authorDisplayName . ‘<br>’;
echo ‘ <strong>Reply:</strong> ‘ . $reply->snippet->textDisplay . ‘<br>’;
}
echo ‘<br>’;
}
}
?>
</body>
</html>
Let me explain the code
function getYouTubeVideoID($url)
This line defines a PHP function named getYouTubeVideoID that takes a URL as its argument. This function extracts the video ID from a YouTube video URL.
$queryString = parse_url($url, PHP_URL_QUERY);
This line uses the parse_url function to parse the URL and extracts the query string part. It then assigns the query string to the variable $queryString.
parse_str($queryString, $params);
This line parses the query string into variables. It assigns the query parameters to an associative array named $params.
if (isset($params[‘v’]) && strlen($params[‘v’]) > 0) {
This line checks if the ‘v’ parameter exists in the $params array and if its length is greater than 0. This ‘v’ parameter typically contains the YouTube video ID.
return $params[‘v’];
If the ‘v’ parameter exists and has a non-zero length, this line returns the value of the ‘v’ parameter, which represents the YouTube video ID.
} else { return “”; }
If the ‘v’ parameter doesn’t exist or has a length of 0, this line returns an empty string.
This line closes the getYouTubeVideoID function definition.
if(isset($_POST[‘submit’])) {
This line checks if the form has been submitted by checking if the ‘submit’ button has been clicked.
$api_key = ‘USE_YOUR_API_KEY‘; // Replace ‘YOUR_API_KEY’ with your actual API key
This line defines the YouTube Data API key. You should replace ‘YOUR_API_KEY’ with your actual API key.
$video_url = $_POST[‘video_url’];
This line retrieves the YouTube video URL from the form input field.
$video_id = getYouTubeVideoID($video_url);
This line calls the getYouTubeVideoID function to extract the video ID from the YouTube video URL.
$video_api_url = ‘https://www.googleapis.com/youtube/v3/videos?part=snippet%2CcontentDetails%2Cstatistics&id=’ . $video_id . ‘&key=’ . $api_key;
This line constructs the URL to fetch video data from the YouTube Data API, including the video ID and API key.
$comments_api_url = ‘https://www.googleapis.com/youtube/v3/commentThreads?part=snippet&videoId=’ . $video_id . ‘&key=’ . $api_key;
This line constructs the URL to fetch comments associated with the video from the YouTube Data API, including the video ID and API key.
$video_data = json_decode(file_get_contents($video_api_url));
This line retrieves the video data from the YouTube Data API and decodes it from JSON format into a PHP object.
$comments_data = json_decode(file_get_contents($comments_api_url));
This line retrieves the comments data from the YouTube Data API and decodes it from JSON format into a PHP object.
echo ‘<br><strong>Title: </strong>’ . $video_data->items[0]->snippet->title . ‘<br>’;
This line prints the title of the video retrieved from the video data.
echo ‘<strong>publishedAt: </strong>’ . $video_data->items[0]->snippet->publishedAt . ‘<br>’;
This line prints the published date of the video retrieved from the video data.
echo ‘<strong>Duration: </strong>’ . $video_data->items[0]->contentDetails->duration . ‘<br>’;
This line prints the duration of the video retrieved from the video data.
echo ‘<strong>View Count: </strong>’ . $video_data->items[0]->statistics->viewCount . ‘<br>’;
This line prints the view count of the video retrieved from the video data.
foreach ($comments_data->items as $comment) {
This line starts a loop to iterate over each comment retrieved from the comments data.
echo ‘<strong>Author:</strong> ‘ . $comment->snippet->topLevelComment->snippet->authorDisplayName . ‘<br>’;
This line prints the display name of the author of the current comment.
echo ‘<strong>Comment:</strong> ‘ . $comment->snippet->topLevelComment->snippet->textDisplay . ‘<br>’;
This line prints the text of the current comment.
$replies_api_url = ‘https://www.googleapis.com/youtube/v3/comments?part=snippet&parentId=’ . $comment->id . ‘&key=’ . $api_key;
This line constructs the URL to fetch replies to the current comment from the YouTube Data API, including the comment ID and API key.
$replies_data = json_decode(file_get_contents($replies_api_url));
This line retrieves the replies data from the YouTube Data API and decodes it from JSON format into a PHP object.
foreach ($replies_data->items as $reply) {
This line starts a loop to iterate over each reply to the current comment.
echo ‘ <strong>Reply Author:</strong> ‘ . $reply->snippet->authorDisplayName . ‘<br>’;
This line prints the display name of the author of the current reply, indented for visual clarity.
echo ‘ <strong>Reply:</strong> ‘ . $reply->snippet->textDisplay . ‘<br>’;
Leave a Reply
You must be logged in to post a comment.
Leave a Comment