The Ultimate Beginner’s Guide to Laravel Cashier
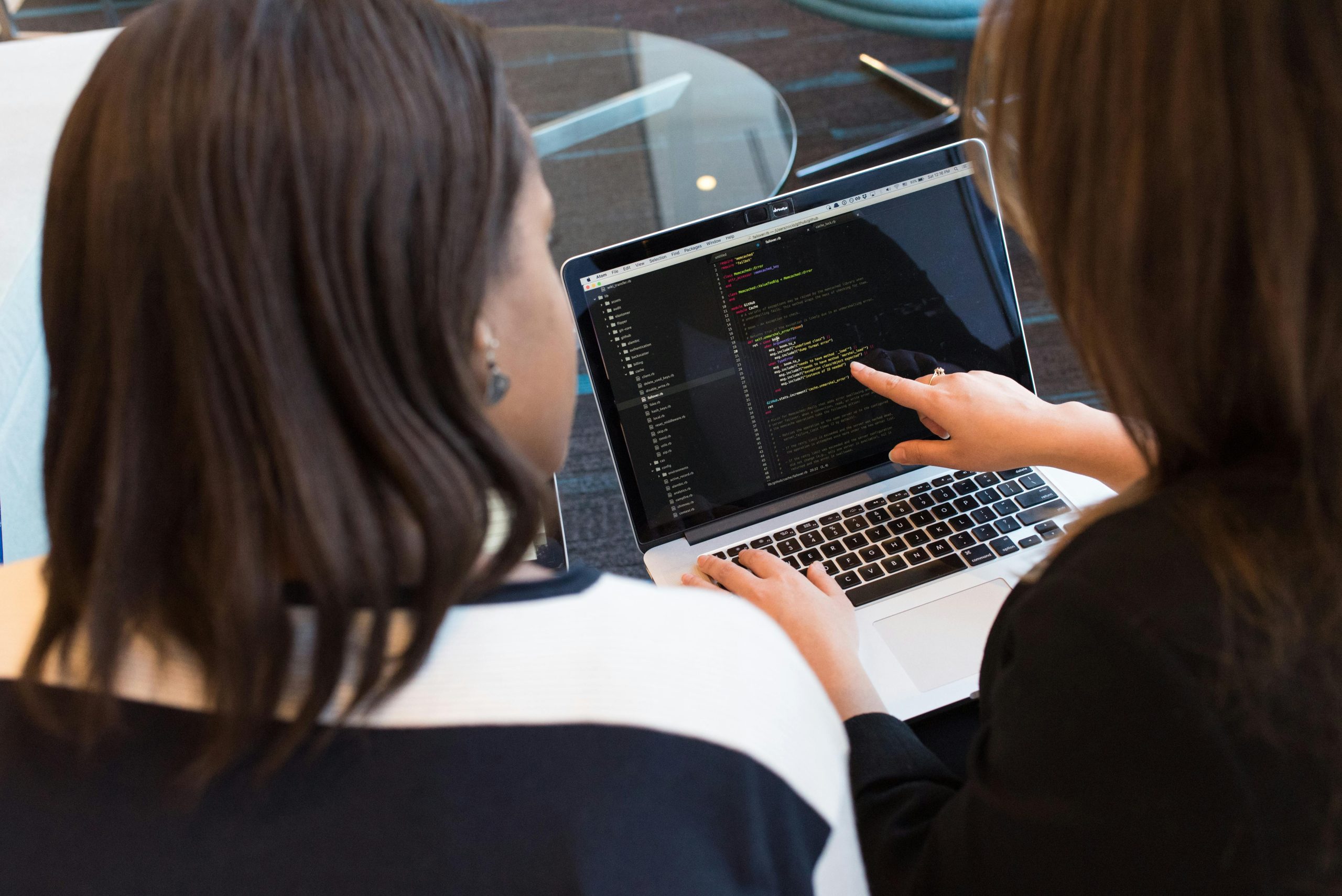
Introduction
If you’re a Laravel developer looking to integrate subscription billing into your application, Laravel Cashier is the perfect tool for you. This powerful package simplifies the process of managing subscriptions with Stripe or Paddle, allowing you to focus on building your application.
In this guide, we’ll walk you through everything you need to know to get started with Laravel Cashier, from installation to managing subscriptions.
What is Laravel Cashier?
Laravel Cashier is a package created by the Laravel team that offers a user-friendly interface to Stripe and Paddle’s subscription billing services. With Cashier, you can easily manage subscription plans, trials, coupons, and payment methods with minimal setup.
Prerequisites
Before we dive into the installation and setup, ensure you have the following:
- A basic understanding of Laravel and PHP.
- Laravel installed on your development machine.
- A Stripe or Paddle account.
Installation
Step 1: Install Laravel Cashier
To get started, you’ll need to install the Laravel Cashier package via Composer. Open your terminal and navigate to your Laravel project directory, then run the following
- composer require laravel/cashier
Step 2: Configuration
After installing Cashier, you need to configure it to work with your application.
- Publish Configuration: First, publish the Cashier configuration file:
php artisan vendor:publish –tag=”cashier-config”
- Environment Variables: Next, add your Stripe or Paddle credentials to your .env file:
STRIPE_KEY=your-stripe-key
STRIPE_SECRET=your-stripe-secret
Migration: Run the migrations to create the necessary database tables for Cashier:
php artisan migrate
Step 3: Billable Model
Laravel Cashier requires you to add the Billable trait to your user model. Open app/Models/User.php and add the trait:
use Laravel\Cashier\Billable;
class User extends Authenticatable
{
use Billable;
}
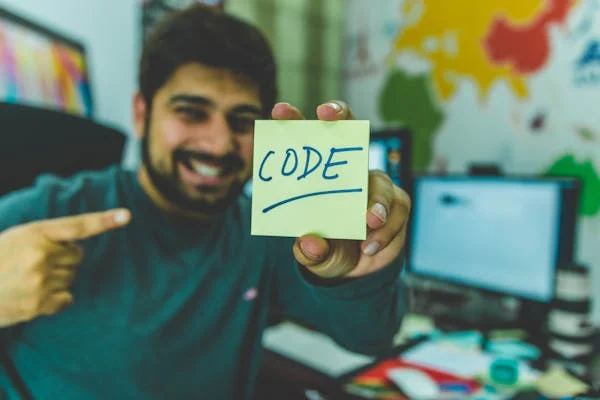
Setting Up Subscriptions
Step 1: Create a Stripe Plan
You must create a plan in Stripe before you can create subscriptions. Sign in to your Stripe dashboard and explore to the “Items” segment. Make another item and add an estimating plan.
Step 2: Creating a Subscription
In order to establish a subscription, the initial step involves creating a form that enables users to input their payment information. Cashier offers a straightforward approach to manage this process by integrating with Stripe’s Checkout.
- Route and Controller: Add a route and controller method for displaying the checkout form:
- Route::get(‘subscribe’, [SubscriptionController::class, ‘showSubscriptionForm’]);
- Route::post(‘subscribe’, [SubscriptionController::class, ‘processSubscription’]);
Subscription Form: Create the subscription form view (resources/views/subscribe.blade.php
):
<form action=”/subscribe” method=”POST”>
@csrf
<input type=”text” name=”plan” placeholder=”Enter Plan ID” required>
<button type=”submit”>Subscribe</button>
</form>
Processing the Subscription: Handle the subscription process in your controller (app/Http/Controllers/SubscriptionController.php
):
public function showSubscriptionForm()Managing Subscriptions
Checking Subscription Status
You can easily check if a user is subscribed to a given plan using Cashier’s built-in methods:
Managing Subscriptions
Checking Subscription Status
You can easily check if a user is subscribed to a given plan using Cashier’s built-in methods:
{
return view(‘subscribe’);
}
public function processSubscription(Request $request)
{
$user = Auth::user();
$paymentMethod = $request->paymentMethod;
$user->newSubscription(‘default’, $request->plan)
->create($paymentMethod);
return redirect(‘/home’)->with(‘success’, ‘Subscription created successfully!’);
}
Managing Subscriptions
Checking Subscription Status
You can easily check if a user is subscribed to a given plan using Cashier’s built-in methods:
if ($user->subscribed(‘default’)) {
// User is subscribed to the default plan
}
Cancelling a Subscription
To cancel a subscription, you can use the cancel method:
$user->subscription(‘default’)->cancel();
Resuming a Subscription
You can use the resume method if a user cancels their subscription and wishes to resume it before the billing period ends:
$user->subscription(‘default’)->resume();
Handling Invoices
Cashier also makes it easy to retrieve and display invoices:
$invoices = $user->invoices();
foreach ($invoices as $invoice) {
echo $invoice->amount();
}
Conclusion
Laravel Clerk is a staggeringly amazing asset for overseeing membership charging in your Laravel applications. By following this aide, you ought to now have a strong comprehension of how to introduce, design, and use Laravel Clerk to deal with memberships easily. Whether you’re fabricating a straightforward application or a complex SaaS stage, Clerk gives the adaptability and usefulness you want to productively deal with your charging.
Leave a Reply
You must be logged in to post a comment.
Leave a Comment