A Comprehensive Guide to Laravel Cashier and Stripe Integration
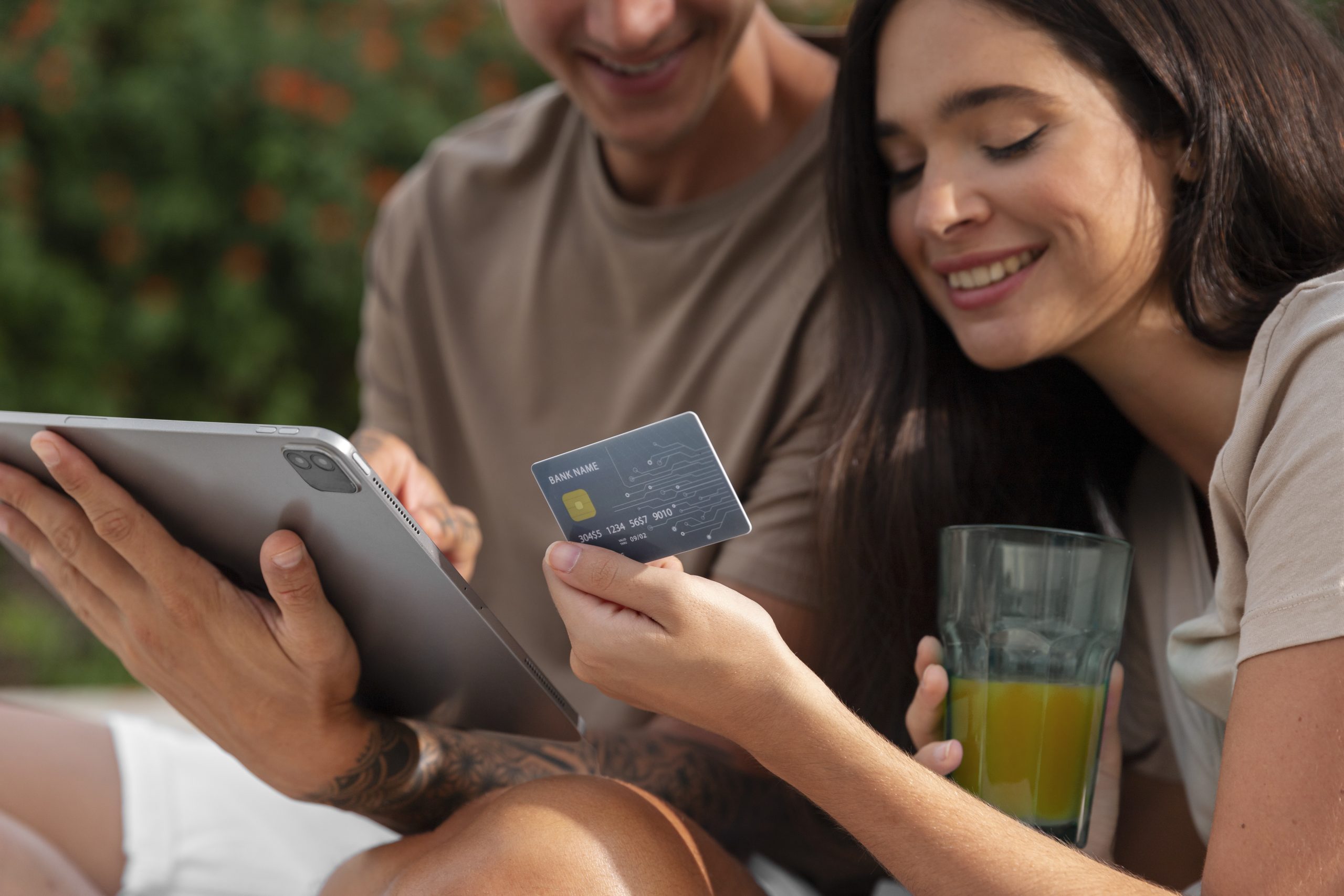
Creating a Stripe developer account is a straightforward process. Follow these steps to set up your account:
1. Visit the Stripe Website: Go to the Stripe website at (https://stripe.com).
2. Sign Up: Click on the “Sign up” button, typically found at the top right corner of the homepage.3. Enter Your Email: Enter your email address in the provided field and click “Continue”.
4. Create an Account: Fill out the form with your full name, a password and other required information. Click “Create account”.
5. Verify Your Email: Check your email for a verification message from Stripe. Click the verification link in the email to verify your email address.
6. Provide Business Details: Once your email is verified, you’ll be prompted to provide more information about your business. If you don’t have a business yet, you can provide general information and update it later.
7. Select Development Environment: You will be taken to the Stripe Dashboard. By default, you’ll be in the “Test” environment. This allows you to test the integration without making real transactions.
8. Obtain API Keys: In the dashboard, navigate to the “Developers” section and select “API keys”. Here you will find your test API keys (Publishable key and Secret key) which you can use to integrate Stripe with your application in a development environment.
9. Set Up Your Account: Complete additional setup steps as required, such as adding a phone number for 2-factor authentication, setting up your banking information (for live transactions), and configuring your account settings.
10. Explore the Documentation: Stripe provides extensive documentation and guides for various programming languages and frameworks. Visit the [Stripe Docs](https://stripe.com/docs) to learn how to integrate Stripe with your application.
11. Test Your Integration: Use the test API keys to simulate transactions and ensure your integration works as expected. Stripe provides test card numbers that you can use to test different scenarios.
12. Go Live: When you are ready to go live, switch to the “Live” environment in the Stripe Dashboard and replace the test API keys with the live API keys in your application.
By following these steps, you’ll have a Stripe developer account set up and be ready to start integrating Stripe’s payment processing capabilities into your application. If you encounter any issues, Stripe’s support and documentation are excellent resources to help you resolve them.
Install Laravel Project using CMD
composer create-project laravel/laravel:^10.0 project-name
If you have to face an error to create DB
Open file: app/Providers/AppServiceProvider.php
And paste this
<?php
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
use Illuminate\Support\Facades\Schema;
class AppServiceProvider extends ServiceProvider
{
/**
* Register any application services.
*/
public function register(): void
{
//
}
/**
* Bootstrap any application services.
*/
public function boot(): void
{
Schema::defaultStringLength(191);
}
}
Follow the Following Steps for Laravel Cashier Stripe Integration
Required Blade Files:
- home.blade.php
- plans.blade.php
- subscription_success.blade.php
- subscription.blade.php
Run Below CMDs:
- composer create-project laravel/laravel:^10.0 example-app
- composer require laravel/ui
- php artisan ui bootstrap –auth
- npm install
- npm run build
- npm run dev
- composer require laravel/cashier
- php artisan vendor:publish –tag=”cashier-migrations”
- php artisan migrate
Models:
- php artisan make:migration create_plans_table
- php artisan make:model Plan
- php artisan make:controller PlanController
- php artisan make:seeder PlanSeeder
- php artisan db:seed –class=PlanSeeder
- php artisan migrate
- php artisan serve
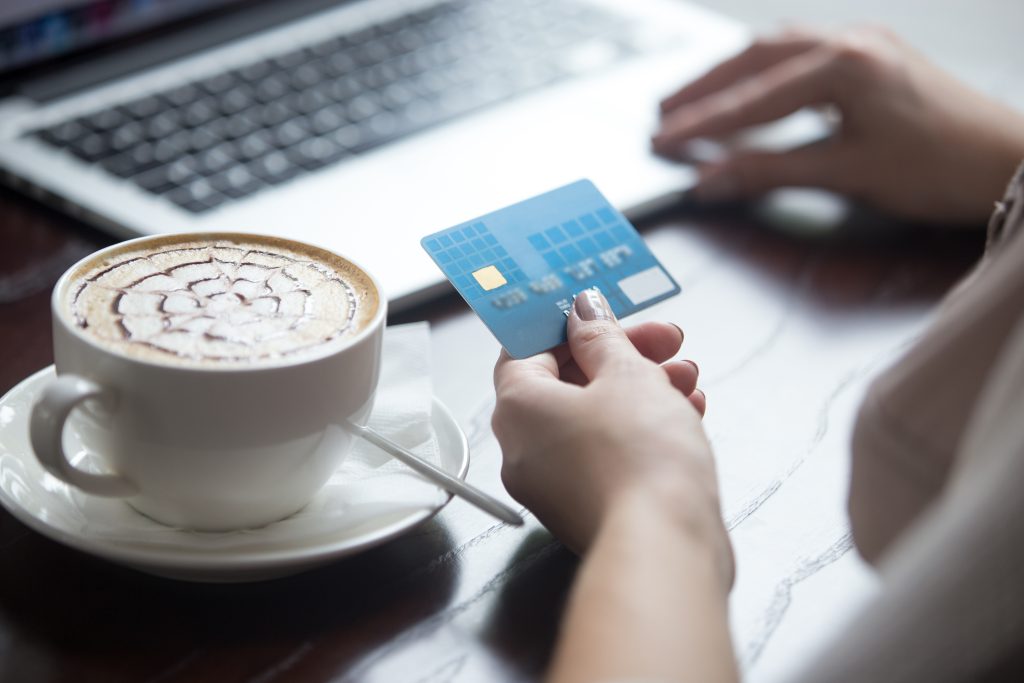
Add these stripe keys (STRIPE_KEY & STRIPE_SECRET) in .ENV fi
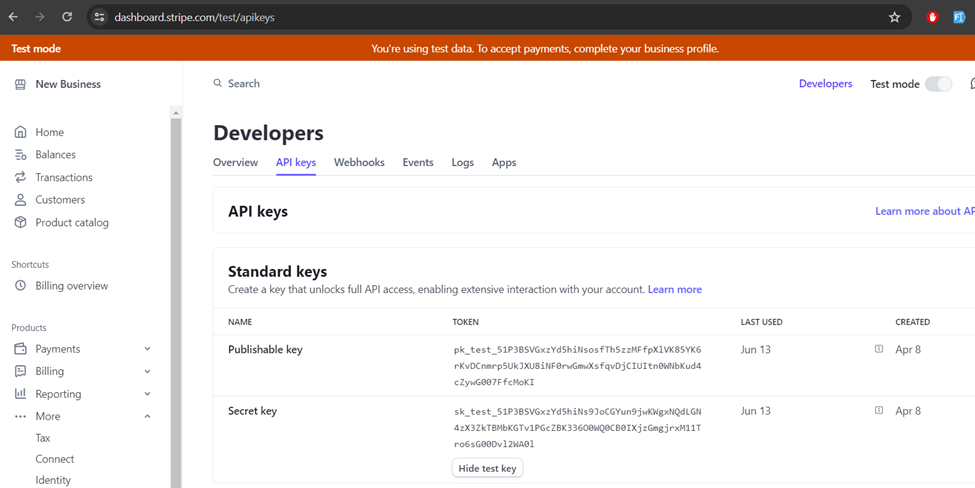
Use Your Stripe Keyz
Required Blade Files:
home.blade.php
@extends(‘layouts.app’)
@section(‘content’)
<div class=”container”>
<div class=”row justify-content-center”>
<div class=”col-md-8″>
<div class=”card”>
<div class=”card-header”>{{ __(‘Dashboard’) }}</div>
<div class=”card-body”>
@if (session(‘status’))
<div class=”alert alert-success” role=”alert”>
{{ session(‘status’) }}
</div>
@endif
{{ __(‘You are logged in!’) }}
</div>
</div>
</div>
</div>
</div>
@endsection
plans.blade.php
@extends(‘layouts.app’)
@section(‘content’)
<div class=”container”>
<section>
<div class=”container py-5″>
<header class=”text-center mb-5 text-white”>
<div class=”row”>
<div class=”col-lg-12 mx-auto”>
<h1>Laravel 9 Cashier Stripe Subscription</h1>
<h3>PRICING</h3>
</div>
</div>
</header>
<div class=”row text-center align-items-end”>
<div class=”col-lg-4 mb-5 mb-lg-0″>
<div class=”bg-white p-5 rounded-lg shadow”>
<h1 class=”h6 text-uppercase font-weight-bold mb-4″>FREE</h1>
<h2 class=”h1 font-weight-bold”>$0<span class=”text-small font-weight-normal ml-2″>/ free</span></h2>
<div class=”custom-separator my-4 mx-auto bg-primary”></div>
<ul class=”list-unstyled my-5 text-small text-left”>
<li class=”mb-3″>
<i class=”fa fa-check mr-2 text-primary”></i> Shared Hosting</li>
<li class=”mb-3″>
<i class=”fa fa-check mr-2 text-primary”></i> Free Web Temp</li>
<li class=”mb-3″>
<i class=”fa fa-check mr-2 text-primary”></i> Free & Easy Site Migration</li>
<li class=”mb-3 text-muted”>
<i class=”fa fa-times mr-2″></i>
<del>100-GB SSD Storage</del>
</li>
<li class=”mb-3 text-muted”>
<i class=”fa fa-times mr-2″></i>
<del>1-Free web domain</del>
</li>
<li class=”mb-3 text-muted”>
<i class=”fa fa-times mr-2″></i>
<del> Free Automatic Backups</del>
</li>
</ul>
<a href=”#” class=”btn btn-primary btn-block shadow rounded-pill”>Buy Now</a>
</div>
</div>
@foreach($plans as $plan)
<div class=”col-lg-4 mb-5 mb-lg-0″>
<div class=”bg-white p-5 rounded-lg shadow”>
<h1 class=”h6 text-uppercase font-weight-bold mb-4″>{{ $plan->name }}</h1>
<h2 class=”h1 font-weight-bold”>${{ $plan->price }}<span class=”text-small font-weight-normal ml-2″>/ month</span></h2>
<div class=”custom-separator my-4 mx-auto bg-primary”></div>
<ul class=”list-unstyled my-5 text-small text-left font-weight-normal”>
<li class=”mb-3″>
<i class=”fa fa-check mr-2 text-primary”></i> Shared Hosting</li>
<li class=”mb-3″>
<i class=”fa fa-check mr-2 text-primary”></i> Free Web Temp </li>
<li class=”mb-3″>
<i class=”fa fa-check mr-2 text-primary”></i> Free & Easy Site Migration </li>
<li class=”mb-3″>
<i class=”fa fa-check mr-2 text-primary”></i100-GB SSD Storage </li>
<li class=”mb-3″>
<i class=”fa fa-check mr-2 text-primary”></i> Free Automatic Backups</li>
<li class=”mb-3 text-muted”>
<i class=”fa fa-times mr-2″></i>
<del>1-Free Domain </del>
</li>
</ul>
<a href=”{{ route(‘plans.show’, $plan->slug) }}” class=”btn btn-primary btn-block shadow rounded-pill”>Buy Now</a>
</div>
</div>
@endforeach
</div>
</div>
</section>
</div>
@endsection
subscription_success.blade.php
@extends(‘layouts.app’)
@section(‘content’)
<div class=”container”>
<div class=”row justify-content-center”>
<div class=”col-md-8″>
<div class=”card”>
<div class=”card-body”>
<div class=”alert alert-success”>
Subscription purchase successfully!
</div>
</div>
</div>
</div>
</div>
</div>
@endsection
subscription.blade.php
@extends(‘layouts.app’)
@section(‘content’)
<div class=”container”>
<div class=”row justify-content-center”>
<div class=”col-md-8″>
<div class=”card”>
<div class=”card-header”>
You will be charged ${{ number_format($plan->price, 2) }} for {{ $plan->name }} Plan
</div>
<div class=”card-body”>
<form id=”payment-form” action=”{{ route(‘subscription.create’) }}” method=”POST”>
@csrf
<input type=”hidden” name=”plan” id=”plan” value=”{{ $plan->id }}”>
<div class=”row”>
<div class=”col-xl-4 col-lg-4″>
<div class=”form-group”>
<label for=””>Name</label>
<input type=”text” name=”name” id=”card-holder-name” class=”form-control” value=”” placeholder=”Name on the card”>
</div>
</div>
</div>
<div class=”row”>
<div class=”col-xl-4 col-lg-4″>
<div class=”form-group”>
<label for=””>Card details</label>
<div id=”card-element”></div>
</div>
</div>
<div class=”col-xl-12 col-lg-12″>
<hr>
<button type=”submit” class=”btn btn-primary” id=”card-button” data-secret=”{{ $intent->client_secret }}”>Purchase</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
<script src=”https://js.stripe.com/v3/”></script>
<script>
const stripe = Stripe(‘{{ env(‘STRIPE_KEY’) }}’)
const elements = stripe.elements()
const cardElement = elements.create(‘card’)
cardElement.mount(‘#card-element’)
const form = document.getElementById(‘payment-form’)
const cardBtn = document.getElementById(‘card-button’)
const cardHolderName = document.getElementById(‘card-holder-name’)
form.addEventListener(‘submit’, async (e) => {
e.preventDefault()
cardBtn.disabled = true
const { setupIntent, error } = await stripe.confirmCardSetup(
cardBtn.dataset.secret, {
payment_method: {
card: cardElement,
billing_details: {
name: cardHolderName.value
}
}
}
)
if(error) {
cardBtn.disable = false
} else {
let token = document.createElement(‘input’)
token.setAttribute(‘type’, ‘hidden’)
token.setAttribute(‘name’, ‘token’)
token.setAttribute(‘value’, setupIntent.payment_method)
form.appendChild(token)
form.submit();
}
})
</script>
@endsection
Run Below CMDs:
- composer create-project laravel/laravel:^10.0 project-name
- composer require laravel/ui
- php artisan ui bootstrap –auth
- npm install
- npm run build
- npm run dev
- composer require laravel/cashier
- php artisan vendor:publish –tag=”cashier-migrations”
- php artisan migrate
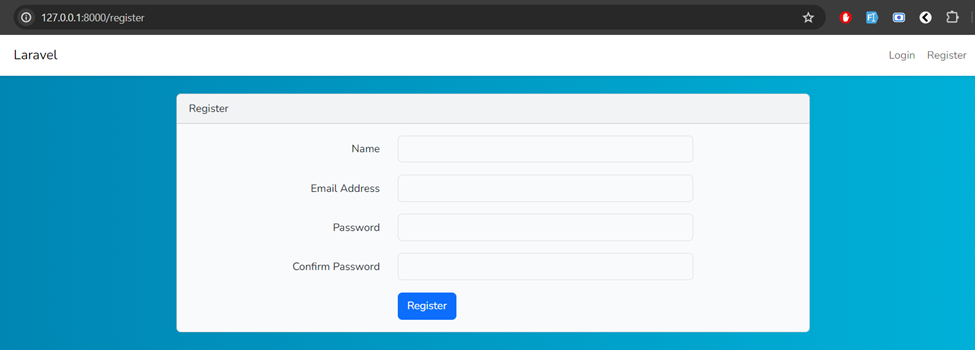
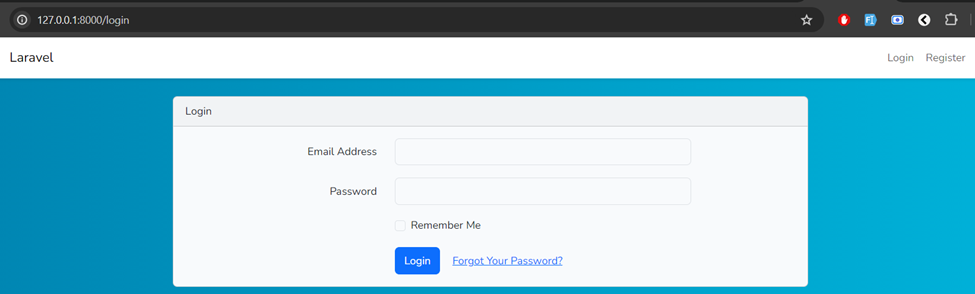
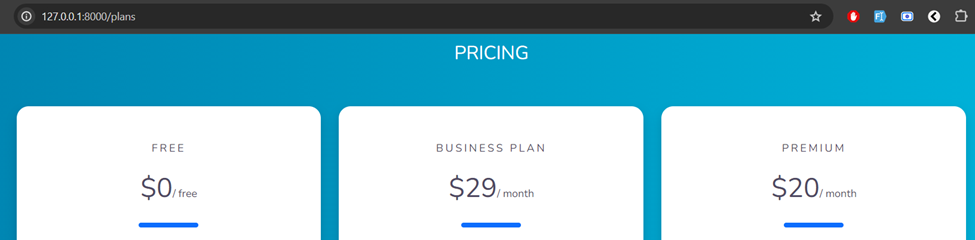
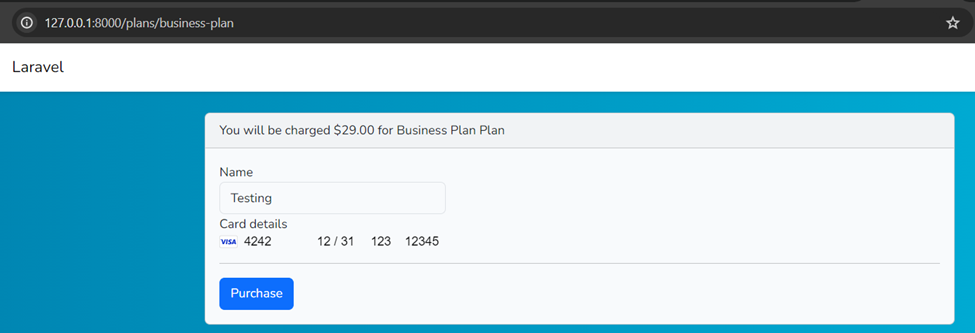
Models:
php artisan make:migration create_plans_table
Schema::create(‘plans’, function (Blueprint $table) {
$table->id();
$table->string(‘name’);
$table->string(‘slug’);
$table->string(‘stripe_plan’);
$table->integer(‘price’);
$table->string(‘description’);
$table->timestamps();
});
php artisan make:model Plan
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Plan extends Model
{
use HasFactory;
protected $fillable = [
‘name’,
‘slug’,
‘stripe_plan’,
‘price’,
‘description’,
];
public function getRouteKeyName()
{
return ‘slug’;
}
}
php artisan make:controller PlanController
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Plan;
class PlanController extends Controller
{
/**
* Write code on Method
*
* @return response()
*/
public function index()
{
$plans = Plan::get();
return view(“plans”, compact(“plans”));
}
/**
* Write code on Method
*
* @return response()
*/
public function show(Plan $plan, Request $request)
{
$intent = auth()->user()->createSetupIntent();
return view(“subscription”, compact(“plan”, “intent”));
}
/**
* Write code on Method
*
* @return response()
*/
public function subscription(Request $request)
{
$plan = Plan::find($request->plan);
$subscription = $request->user()->newSubscription($request->plan, $plan->stripe_plan)
->create($request->token);
return view(“subscription_success”);
}
}
php artisan make:seeder PlanSeeder
(Make Sure your stripe-plan key-id is same as copy from the strip subscription account)
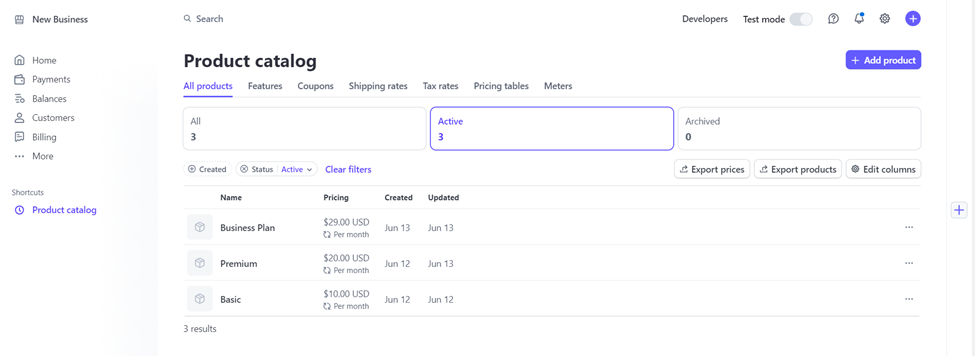
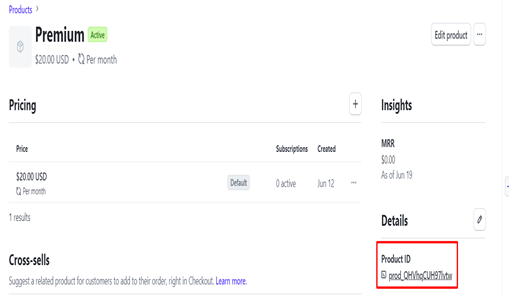
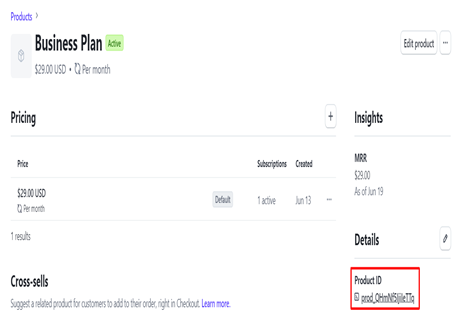
<?php
namespace Database\Seeders;
use Illuminate\Database\Seeder;
use App\Models\Plan;
class PlanSeeder extends Seeder
{
public function run()
{
$plans = [
[
‘name’ => ‘Business Plan’,
‘slug’ => ‘business-plan’,
‘stripe_plan’ => ‘price_1PRCp2GxzYd5hiNsSzX8h1aC’,
‘price’ => 29,
‘description’ => ‘Business Plan’
],
[
‘name’ => ‘Premium’,
‘slug’ => ‘premium’,
‘stripe_plan’ => ‘price_1PQwgCGxzYd5hiNsKYbDIDfy’,
‘price’ => 20,
‘description’ => ‘Premium’
]
];
foreach ($plans as $plan) {
Plan::create($plan);
}
}
}
Routes/web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\PlanController;
Route::get(‘/’, function () {
return view(‘welcome’);
});
Auth::routes();
Route::get(‘/home’, [App\Http\Controllers\HomeController::class, ‘index’])->name(‘home’);
Route::middleware(“auth”)->group(function () {
Route::get(‘plans’, [PlanController::class, ‘index’]);
Route::get(‘plans/{plan}’, [PlanController::class, ‘show’])->name(“plans.show”);
Route::post(‘subscription’, [PlanController::class, ‘subscription’])->name(“subscription.create”);
});
Inculude this if having an error related to bill
use Laravel\Cashier\Billable;
class User extends Authenticatable
{
use HasApiTokens, HasFactory, Notifiable, Billable;
}
- php artisan db:seed –class=PlanSeeder
- php artisan migrate
- php artisan serve
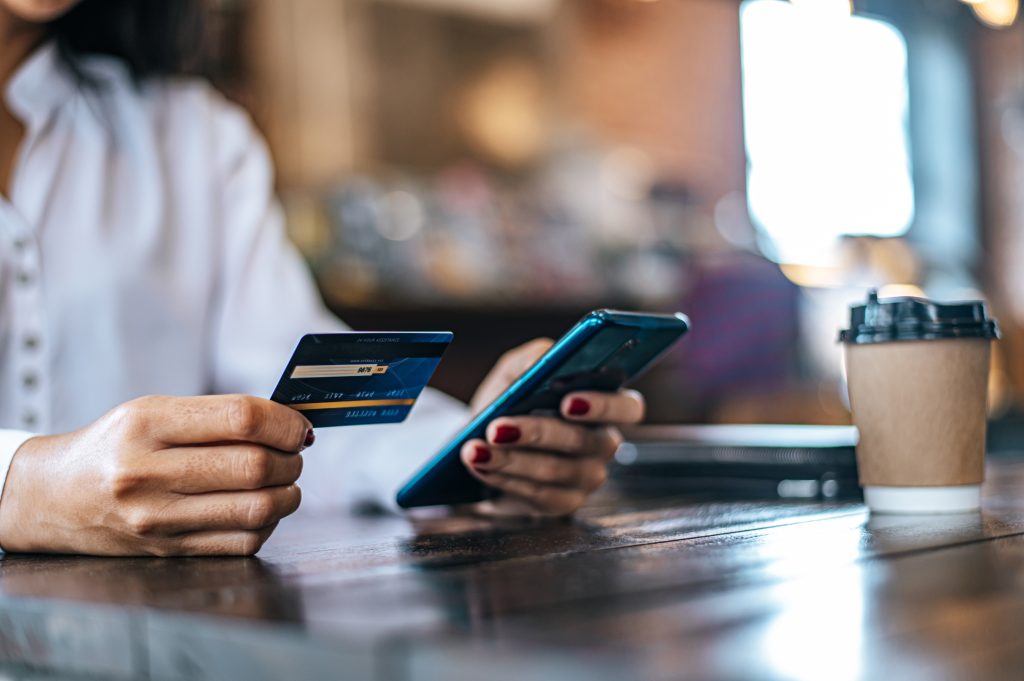
Leave a Reply
You must be logged in to post a comment.
Leave a Comment